반응형
Gson은 JSON 직렬화 및 역직렬화에서 유연한 필드 제어를 제공합니다. @Expose, @SerializedName, 리플렉션 등의 기능을 활용하여 개발 중 발생할 수 있는 다양한 요구사항을 손쉽게 해결할 수 있습니다.
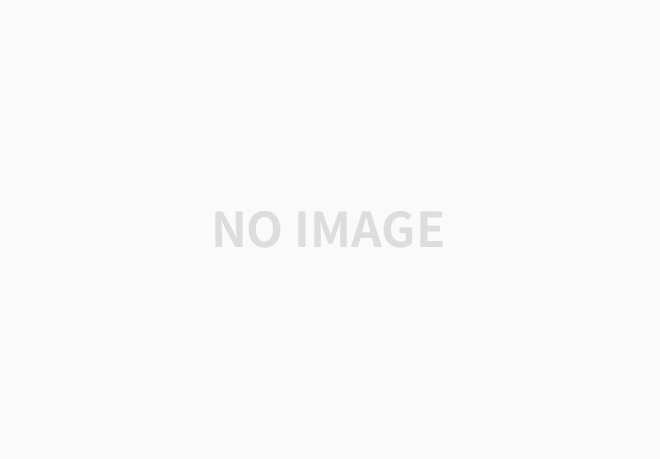
특정 필드 제외하기 (@Expose, excludeFieldsWithoutExposeAnnotation)
Gson은 기본적으로 모든 필드를 JSON에 포함합니다. 하지만 필요에 따라 특정 필드를 제외할 수 있습니다. 이를 위해 @Expose 애너테이션과 excludeFieldsWithoutExposeAnnotation 옵션을 사용할 수 있습니다.
@Expose를 활용한 특정 필드 제외
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.annotations.Expose;
public class Main {
public static void main(String[] args) {
// 객체 생성
User user = new User("박민수", 30, "비밀정보");
// Gson 객체 생성
Gson gson = new GsonBuilder()
.excludeFieldsWithoutExposeAnnotation()
.setPrettyPrinting()
.create();
// 직렬화
String json = gson.toJson(user);
System.out.println("직렬화된 JSON:");
System.out.println(json);
}
}
class User {
@Expose
String name;
@Expose
int age;
String sensitiveData; // 제외됨
public User(String name, int age, String sensitiveData) {
this.name = name;
this.age = age;
this.sensitiveData = sensitiveData;
}
}
출력 결과:
직렬화된 JSON:
{
"name": "박민수",
"age": 30
}
위 예제에서는 sensitiveData 필드가 @Expose 애너테이션이 없으므로 직렬화에서 제외되었습니다.
반응형
JSON 필드 이름 커스터마이징 (@SerializedName)
JSON 필드 이름을 클래스 필드와 다르게 정의해야 할 때 @SerializedName 애너테이션을 사용할 수 있습니다. 이를 통해 JSON 필드 이름과 클래스 필드 이름을 매핑할 수 있습니다.
@SerializedName을 활용한 필드 이름 매핑
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.annotations.SerializedName;
public class Main {
public static void main(String[] args) {
// 객체 생성
Person person = new Person("김영희", "서울특별시", "개발팀");
// Gson 객체
Gson gson = new GsonBuilder().setPrettyPrinting().create();
// 직렬화
String json = gson.toJson(person);
System.out.println("직렬화된 JSON:");
System.out.println(json);
// 역직렬화
String inputJson = "{\"full_name\":\"김영희\",\"city\":\"서울특별시\",\"department\":\"개발팀\"}";
Person deserializedPerson = gson.fromJson(inputJson, Person.class);
System.out.println("\n역직렬화된 객체:");
System.out.println("이름: " + deserializedPerson.fullName);
System.out.println("도시: " + deserializedPerson.city);
System.out.println("부서: " + deserializedPerson.department);
}
}
class Person {
@SerializedName("full_name")
String fullName;
@SerializedName("city")
String city;
@SerializedName("department")
String department;
public Person(String fullName, String city, String department) {
this.fullName = fullName;
this.city = city;
this.department = department;
}
}
출력 결과:
직렬화된 JSON:
{
"full_name": "김영희",
"city": "서울특별시",
"department": "개발팀"
}
역직렬화된 객체:
이름: 김영희
도시: 서울특별시
부서: 개발팀
@SerializedName은 필드 이름이 복잡한 API와 상호작용할 때 매우 유용합니다.
접근 제한 필드 처리
Gson은 기본적으로 public 필드만 처리합니다. 하지만 private 필드도 포함하려면 리플렉션을 사용하거나 Gson의 전략을 변경할 수 있습니다.
리플렉션을 활용한 private 필드 처리
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
public class Main {
public static void main(String[] args) {
// 객체 생성
Employee employee = new Employee("이철수", "인천광역시", "마케팅팀");
// Gson 객체
Gson gson = new GsonBuilder()
.excludeFieldsWithoutExposeAnnotation()
.setPrettyPrinting()
.create();
// 직렬화
String json = gson.toJson(employee);
System.out.println("직렬화된 JSON:");
System.out.println(json);
}
}
class Employee {
@Expose
private String name;
@Expose
private String address;
@Expose
private String department;
public Employee(String name, String address, String department) {
this.name = name;
this.address = address;
this.department = department;
}
}
출력 결과:
직렬화된 JSON:
{
"name": "이철수",
"address": "인천광역시",
"department": "마케팅팀"
}
위 예제에서는 private 필드도 @Expose 애너테이션을 활용하여 JSON에 포함되었습니다.
Gson 필드 컨트롤의 활용 사례
- 특정 필드 제외는 보안이 중요한 데이터(예: 비밀번호, 토큰 등)를 처리할 때 유용합니다.
- JSON 필드 이름 커스터마이징은 외부 API와 JSON 형식을 맞출 때 효율적입니다.
- 접근 제한 필드 처리는 엔티티 클래스의 캡슐화를 유지하면서도 JSON 데이터를 직렬화할 때 활용됩니다.
참고사이트
Gson 공식 문서: https://github.com/google/gson
반응형
'JAVA' 카테고리의 다른 글
[JAVA] Gson의 제한사항과 문제 해결 (0) | 2024.12.14 |
---|---|
[JAVA] Gson을 활용한 성능 최적화 (0) | 2024.12.14 |
[JAVA] Gson과 JSON 파싱 (0) | 2024.12.14 |
[JAVA] Gson과 날짜/시간 처리 (0) | 2024.12.14 |
[JAVA] Gson과 복합 객체 (0) | 2024.12.14 |
[JAVA] Gson의 고급 기능 (1) | 2024.12.14 |
[JAVA] Gson과 Java 컬렉션 (0) | 2024.12.13 |
[JAVA] Gson을 활용한 기본 JSON 처리 (0) | 2024.11.25 |