Gson과 REST API는 JSON 데이터 포맷을 주고받는 REST API와 Gson을 활용하여 데이터를 처리하는 방법에 초점을 맞춥니다. REST API와 통합하여 효율적으로 데이터를 직렬화 및 역직렬화하고, Retrofit이나 Spring Boot와 같은 프레임워크와 함께 사용하는 방법을 예제를 통해 설명합니다.
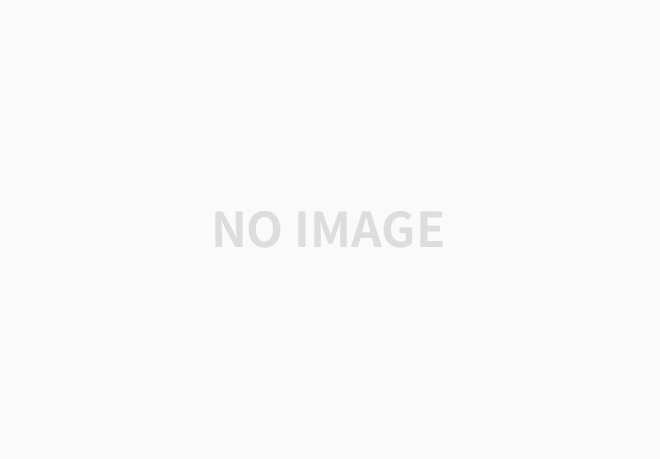
REST API와의 통합
Gson은 REST API와의 통합에서 JSON 데이터의 요청(Request)과 응답(Response)을 처리하는 데 중요한 역할을 합니다. 서버에서 반환된 JSON 데이터를 객체로 변환하거나, 객체를 JSON 형식으로 변환하여 서버에 요청을 보낼 수 있습니다.
import com.google.gson.Gson;
class User {
String name;
int age;
String city;
public User(String name, int age, String city) {
this.name = name;
this.age = age;
this.city = city;
}
}
public class RestApiExample {
public static void main(String[] args) {
// 객체를 JSON으로 변환 (Request)
User user = new User("김영희", 25, "서울");
Gson gson = new Gson();
String jsonRequest = gson.toJson(user);
System.out.println("JSON Request: " + jsonRequest);
// JSON 응답을 객체로 변환 (Response)
String jsonResponse = "{ \"name\": \"박철수\", \"age\": 30, \"city\": \"부산\" }";
User responseUser = gson.fromJson(jsonResponse, User.class);
System.out.println("Response User: " + responseUser.name + ", " + responseUser.age + ", " + responseUser.city);
}
}
Retrofit과 함께 사용하는 방법
Retrofit은 REST API 클라이언트 라이브러리로 Gson을 활용해 JSON 데이터를 간단히 처리할 수 있습니다. Gson을 통합하려면 GsonConverterFactory를 추가하면 됩니다.
import retrofit2.Retrofit;
import retrofit2.converter.gson.GsonConverterFactory;
import retrofit2.Call;
import retrofit2.http.Body;
import retrofit2.http.GET;
import retrofit2.http.POST;
class User {
String name;
int age;
String city;
// 생성자, getter, setter 생략
}
interface ApiService {
@GET("/users")
Call<User> getUser();
@POST("/users")
Call<Void> createUser(@Body User user);
}
public class RetrofitExample {
public static void main(String[] args) {
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://api.example.com")
.addConverterFactory(GsonConverterFactory.create())
.build();
ApiService apiService = retrofit.create(ApiService.class);
// POST 요청 예제
User newUser = new User("홍길동", 27, "대구");
Call<Void> call = apiService.createUser(newUser);
// 실제 네트워크 요청은 비동기로 처리 (enqueue 사용)
}
}
Spring Boot와 함께 사용하는 방법
Spring Boot에서는 기본적으로 Jackson이 사용되지만, Gson을 대체로 사용할 수도 있습니다. GsonHttpMessageConverter를 설정하여 Gson을 사용할 수 있습니다.
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.converter.json.GsonHttpMessageConverter;
@Configuration
public class GsonConfig {
@Bean
public GsonHttpMessageConverter gsonHttpMessageConverter() {
Gson gson = new GsonBuilder().setPrettyPrinting().create();
return new GsonHttpMessageConverter(gson);
}
}
JSON Request/Response 데이터 변환 예제
Spring Boot 컨트롤러에서 JSON 요청을 Gson으로 처리하는 방법은 다음과 같습니다.
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/users")
public class UserController {
@PostMapping
public String createUser(@RequestBody User user) {
return "사용자가 생성되었습니다: " + user.name + ", " + user.city;
}
@GetMapping("/{name}")
public User getUser(@PathVariable String name) {
return new User(name, 28, "인천");
}
}
Gson의 장점과 REST API에서 활용
- 경량화된 JSON 처리: Gson은 가볍고 직관적이기 때문에 빠른 개발과 디버깅이 가능합니다.
- 객체 변환의 유연성: 복잡한 JSON 구조에서도 쉽게 객체로 변환 가능합니다.
- 프레임워크와의 통합: Retrofit과 Spring Boot와 같은 인기 프레임워크와 자연스럽게 통합됩니다.
참고사이트
- Gson 공식 문서: https://github.com/google/gson
- Retrofit 공식 문서: https://square.github.io/retrofit/
- Spring Boot 공식 문서: https://spring.io/projects/spring-boot
'JAVA' 카테고리의 다른 글
[JAVA] Gson의 확장과 커스터마이징 (1) | 2024.12.15 |
---|---|
[JAVA] Gson과 최신 Java 기능 연계 (0) | 2024.12.15 |
[JAVA] Gson 자주 발생하는 문제와 해결법 (0) | 2024.12.15 |
[JAVA] 실전 프로젝트에서의 Gson 활용 (0) | 2024.12.15 |
[JAVA] Gson의 제한사항과 문제 해결 (0) | 2024.12.14 |
[JAVA] Gson을 활용한 성능 최적화 (0) | 2024.12.14 |
[JAVA] Gson과 JSON 파싱 (0) | 2024.12.14 |
[JAVA] Gson과 날짜/시간 처리 (0) | 2024.12.14 |